Customer Relationship Management (CRM) is a system that manages customer interactions and data throughout the customer lifecycle between the customer and the company across different channels. In this tutorial, we are going to build a custom CRM in PHP, which a sales team can use to track customers through the entire sales cycle.
We’ll be creating a simple CRM system for salespeople to:
- Access their tasks
- View their leads
- Create new tasks for each lead
- Create new opportunity
- Lose a sale
Sales managers will be able to:
- Manage all customers
- Manage sales team
- View current sales activities
Building Blocks of a CRM
Here is a list of the essential components of the CRM:
- Leads: initial contacts
- Accounts: Information about the companies you do business with
- Contact: Information about the people you know and work with. Usually, one account has many contacts
- Opportunities: Qualified leads
- Activities: Tasks, meetings, phone calls, emails and any other activities that allow you to interact with customers
- Sales: Your sales team
- Dashboard: CRM dashboards are much more than just eye candy. They should deliver key information at a glance and provide links to drill down for more details.
- Login: Salespeople and managers have different roles in the system. Managers have access to reports and sales pipeline information.
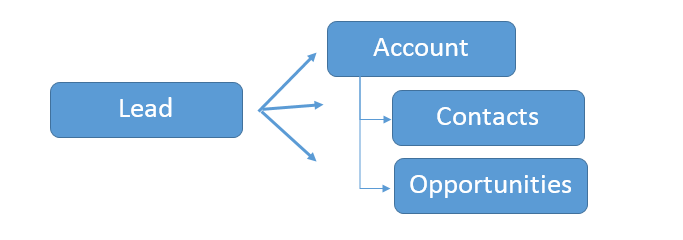
System Requirements
- PHP 7
- MySQL or MariaDB
- phpGrid
Create CRM Database
We will start by creating our custom CRM database. The main tables we will be using are:
- contact – contains basic customer data
- notes – holds information collection from Contact by sales people.
- users – information about sales people
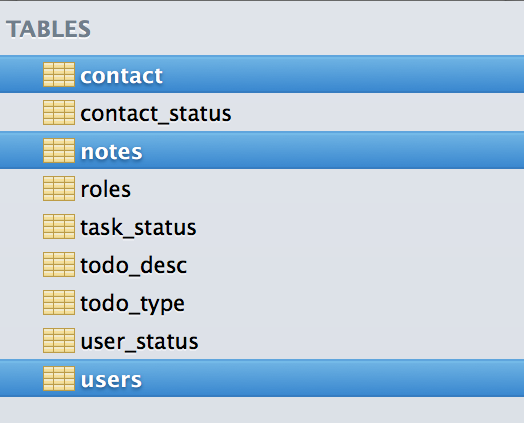
The contact table contains basic customer information including names, company addresses, project information, etc.
The notes table is used to store all sales activity information such as meetings and phone calls.
The users table holds login information about users of the system such as usernames and passwords. Users can also have roles, either Sales or Manager.
All other tables are simple lookup tables to join to the aforementioned three main relational database tables.
- contact_status – contains contact status such as Lead and Opportunity each indicating a different stage in a typical sales cycle
- task_status – the task status can be either Pending or Completed
- user_status – a sale person can be Active or Inactive
- todo_type – a type of task either Task or Meeting
- todo_desc – description of a task such as Follow Up Email, Phone Call, and Conference etc.
- roles – a user can be either a Sales Rep or a Manager
Complete Database Schema Diagram
A database schema is the structure that represents the logical view (tables, views, primary and foreign keys) of the entire database. A database schema includes entities and the relationship among them.
It is a good practice to have one primary key for each table in a relational database. A primary key is a unique identifier for each record. It can be the social security number (SSN), vehicle identification number (VIN), or simply auto-increment number (a unique number that is generated when a new record is inserted into a table).
Below is the database diagram of our simple CRM. The key symbol in each table represents the table primary key. The magnifying glass indicates foreign key linking another table in the database. Sometimes we call it the “lookup” table.
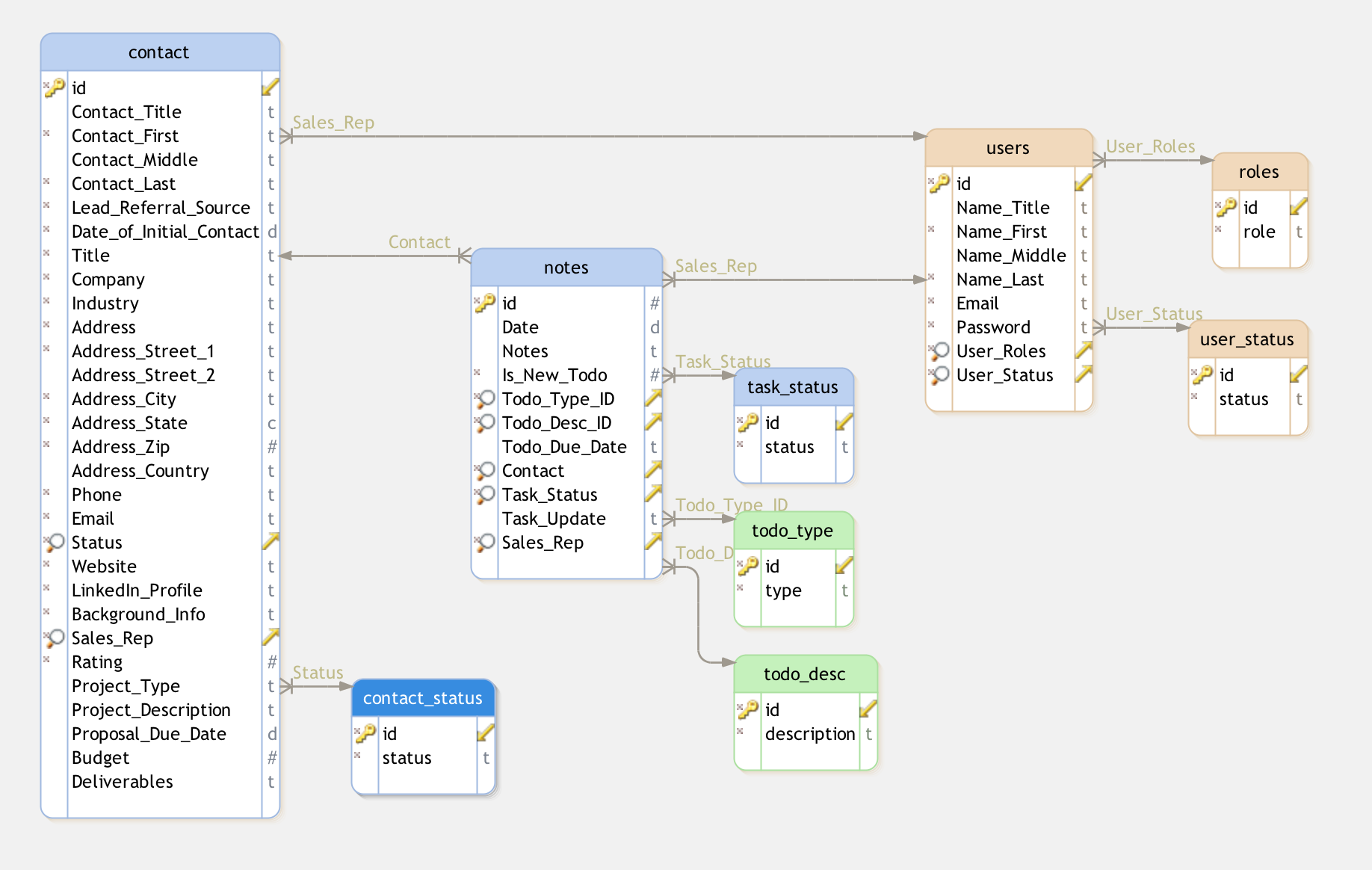
install.sql
Once you have an understanding of the database table structure, find the `install.sql` script in the db folder and use a MySQL tool such as MySQL Workbench or Sequel Pro to run the SQL script. It should create a new relational database named custom_crm and its database tables.
Setup phpGrid
Our simple CRM contains many datagrids. The datagrid is a spreadsheet-like data table that displays rows and columns representing records and fields from the database table. The datagrid gives the end-user ability to read and write to database tables on a web page.
We opt-in a datagrid tool from phpGrid to create the datagrid. The reason to use a tool instead of building them from scratch is that developing the datagrid is usually extremely tedious and error-prone. The datagrid library will handle all internal database CRUD (Create, Remove, Update, and Delete) operations for us with better and faster results with little code.
To install phpGrid, follow these steps:
- Unzip the phpGrid download file
- Upload the phpGrid folder to the `phpGrid` folder
- Complete the installation by configuring the `conf.php` file
Before we begin coding, we must specify the database information in `conf.php`, the phpGrid configuration file. Here is an example of database connection settings:
1 2 3 4 5 6 |
- PHPGRID_DB_HOSTNAME – web server IP or host name
- PHPGRID_DB_USERNAME – database user name
- PHPGRID_DB_PASSWORD – database password
- PHPGRID_DB_NAME – database name of our CRM
- PHPGRID_DB_TYPE – type of database
- PHPGRID_DB_CHARSET – always ‘utf8’ in MySQL
Page Template
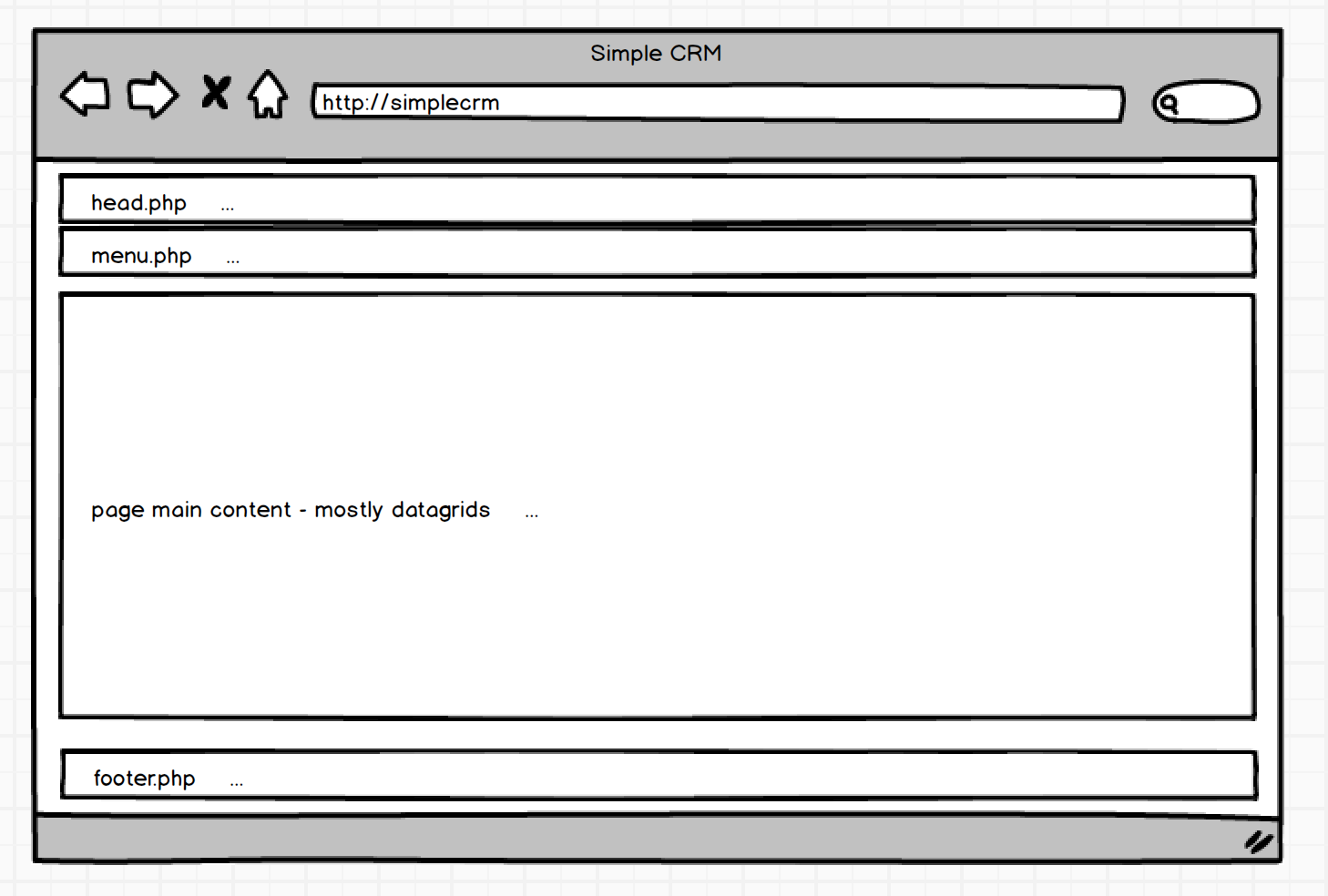
Before we can start building our first page of the CRM, it is a good practice to make the page items reusable such as header and footer.
The page will be comprised of a header, menu, body and footer. We will start by creating a reusable page template.
head.php
This is a basic HTML5 template header. It includes a link to a custom stylesheet that will be created in a later step.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | <!DOCTYPE html> <html lang="en"> <head> <title>My Custom CRM</title> <meta charset="utf-8"> <link rel="stylesheet" href="../css/style.css"> </head> <body></code> </html> <h4>menu.php</h4> <figure id="post-251806" class="align-none media-251806"><img src="https://raw.githubusercontent.com/phpcontrols/phpgrid-custom-crm/master/design/menus/custom-crm-salesrep-menu.png" alt="" /><figcaption>The Top Menu Bar</figcaption></figure> [cc lang="html"] <div id="menu"> <ul> <li><a href="tasks.php" <?php if($_GET['currentPage'] == 'tasks') echo 'class="active"'; ?>>Tasks</a></li> <li><a href="leads.php" <?php if($_GET['currentPage'] == 'leads') echo 'class="active"'; ?>>Leads</a></li> <li><a href="opportunities.php" <?php if($_GET['currentPage'] == 'opportunities') echo 'class="active"'; ?>>Opportunities</a></li> <li><a href="customerwon.php" <?php if($_GET['currentPage'] == 'customerwon') echo 'class="active"'; ?>>Customers/Won</a></li> </ul> </div></code> |
Notice the usage of
1 | $_GET['currentPage'] |
Each page will set a value which will highlight the name of the current page on the top menu bar.
Include the following code in `style.css` for menu styling. It will transform the above, unordered list into a menu.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #menu ul { list-style-type: none; margin: 0; padding: 0; overflow: hidden; background-color: #1590C2; } #menu ul li { float: left; } #menu ul li a { display: block; color: white; text-align: center; padding: 14px 16px; text-decoration: none; } #menu ul li a:hover, #menu .active { background-color: #ddd; color: black; }</code> |
footer.php
Closing tags for the elements we opened in the header:
1 2 | </body> </html> |
Our Complete Reusable Page Template
This is the complete page template. The main content will go after Section Title.
1 2 | include_once("../phpGrid/conf.php"); include_once('../inc/head.php'); |
1 2 3 4 5 6 7 8 9 10 11 | <h1>My Custom CRM</h1> <?php include_once('../inc/menu.php'); ?> <h3>Section Title</h3> <?php // Your main content, such as a datagrid, goes here ?> <?php include_once('../inc/footer.php'); ?> |
CRM Main Pages
Are you still with me? Good! We can now finally develop the first page in our CRM.
Our CRM for sales team members has four pages:
- Tasks
- Leads
- Opportunities
- Customers/Won
Each page indicates a different stage in a typical sales cycle.
Design Mockup
Here’s our CRM design mockup for the sales people.
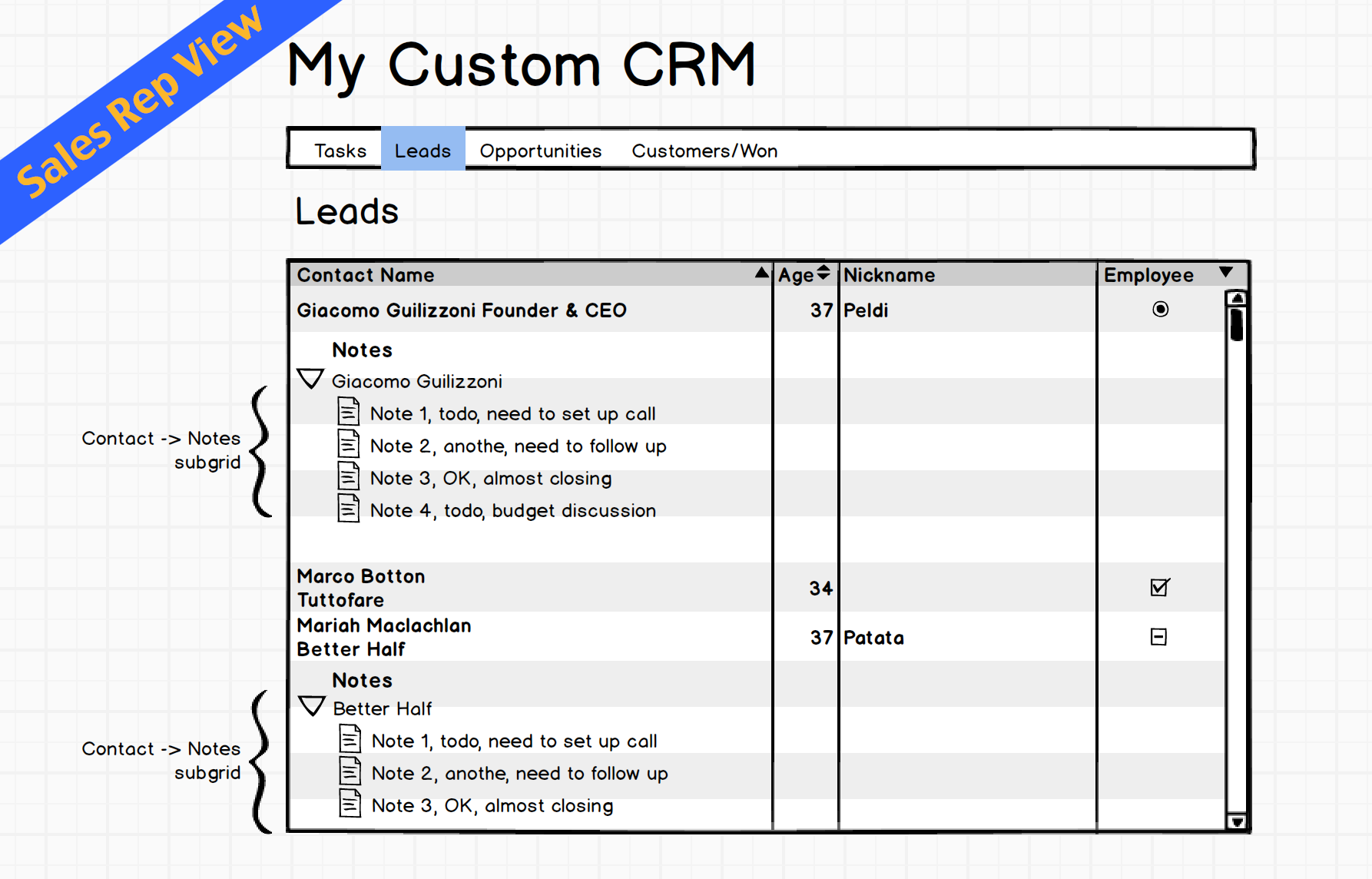
Tasks
When a sales team member logged in, the first page he sees is a list of current tasks.
As you may recall, our notes table holds all the sales activity information. We can create a datagrid and populate it from the Notes table using phpGrid.
The Tasks page main content is a datagrid. The following two lines will give us a list of tasks of the current sales person.
1 2 3 | $dg = new C_DataGrid("SELECT * FROM notes", "ID", "notes"); $dg -> display(); ?> |
- The first line creates a phpGrid object by passing the SELECT SQL statement, its primary key – ID, and then the name of the database table – notes.
- The second and the final line calls display() function to render the datagrid on the screen. Check out the basic datagrid demo for more detail.
Leads
The leads page contains list of current leads that the sales person is responsible for. Each Lead can have one or many Notes. We will use the phpGrid subgrid feature for that.
We also need to use set_query_filter() to display only the leads, Status = 1, and only for the current sales person.
Contact status table
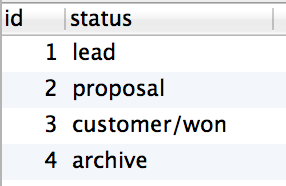
1 2 3 4 5 6 7 8 9 10 | $dg = new C_DataGrid("SELECT * FROM contact", "id", "contact"); $dg->set_query_filter(" Status = 1 && sales_rep = 1 "); // the detail grid displays notes about a lead $sdg = new C_DataGrid("SELECT * FROM notes", "id", "notes"); $sdg->set_query_filter(" Sales_Rep = 1 "); $sdg->enable_edit(); $dg->set_subgrid($sdg, 'Contact', 'id'); $dg -> display(); |
Opportunities
A Lead becomes an Opportunity once it is qualified. The Opportunities page is similar to the Leads page. The only difference is the filtered status code in set_query_filter is Status = 2.
1 2 3 4 5 6 7 8 9 | $dg = new C_DataGrid("SELECT * FROM contact", "id", "contact"); $dg->set_query_filter(" Status = 2 && sales_rep = 1 "); $sdg = new C_DataGrid("SELECT * FROM notes", "id", "notes"); $sdg->set_query_filter(" Sales_Rep = 1 "); $sdg->enable_edit(); $dg->set_subgrid($sdg, 'Contact', 'id'); $dg -> display(); |
Customers/Won
Customers/Won has the Status = 3. Similar to Leads and Opportunities, Customers/Won can also have Notes.
1 2 3 4 5 6 7 8 9 | $dg = new C_DataGrid("SELECT * FROM contact", "id", "contact"); $dg->set_query_filter(" Status = 3 && sales_rep = 1 "); $sdg = new C_DataGrid("SELECT * FROM notes", "id", "notes"); $sdg->set_query_filter(" Sales_Rep = 1 "); $sdg->enable_edit(); $dg->set_subgrid($sdg, 'Contact', 'id'); $dg -> display(); |
That’s all there is to it for sales people in our simple CRM.
Manager Dashboard
The sales manager will have access to all records in the sales pipeline as well as the ability to manage sales team and customer data.
Here’s the design mockups:
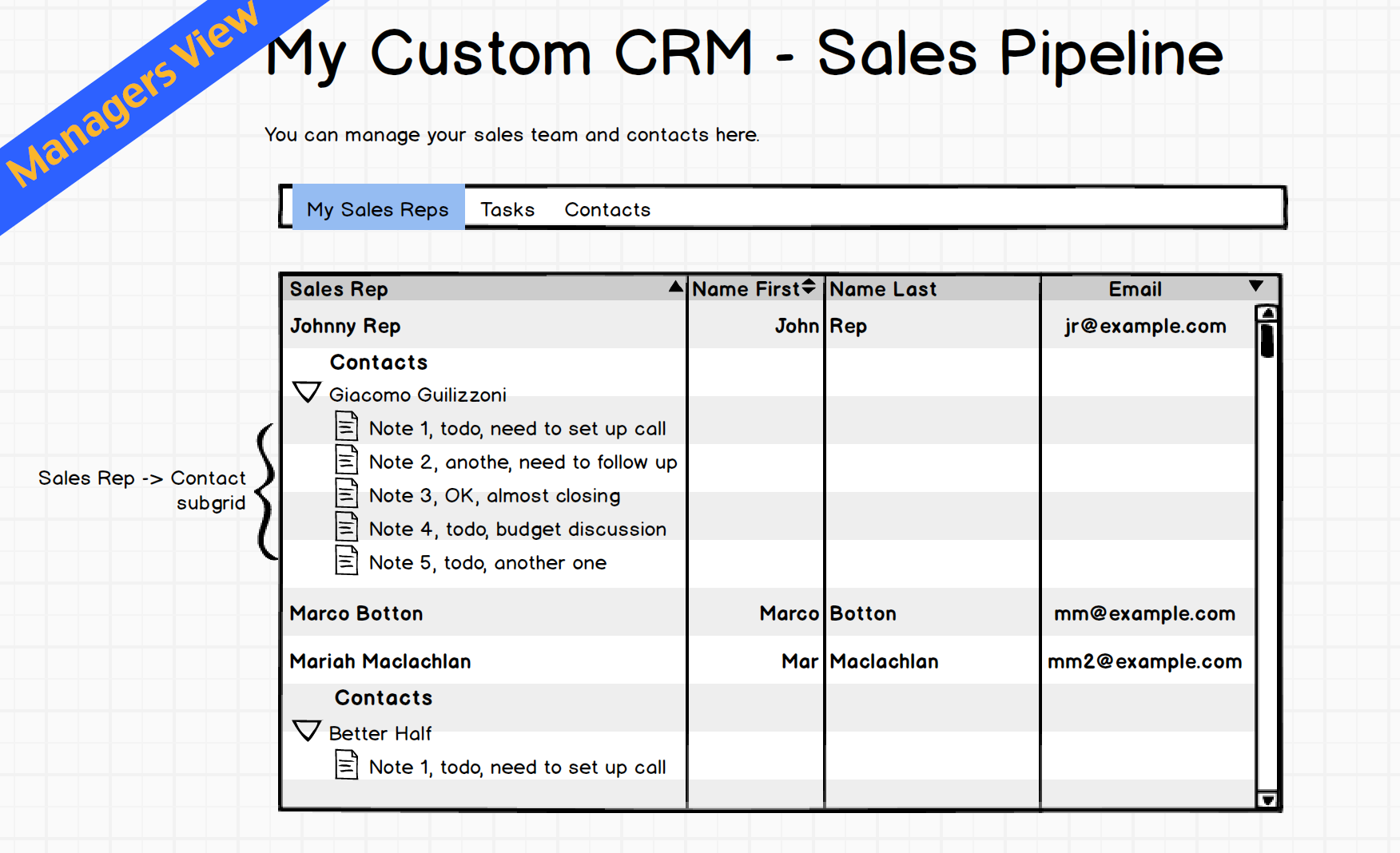
Menu
Managers Dashboard has three menu items.
1 2 3 4 5 6 7 | <div id="menu"> <ul> <li><a href="?gn=users" <?php if($tableName == 'users') echo 'class="active"'; ?>>My Sales Reps</a></li> <li><a href="?gn=notes" <?php if($tableName == 'notes') echo 'class="active"'; ?>>Tasks</a></li> <li><a href="?gn=contact" <?php if($tableName == 'contact') echo 'class="active"'; ?>>Contact</a></li> </ul> </div> |
Main content
Each menu item represents a table in the CRM database. $_GET[‘gn’] will store the table name. It dynamically generates the datagrid based on table name passed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | $tableName = (isset($_GET['gn']) && isset($_GET['gn']) !== '') ? $_GET['gn'] : 'users'; switch($tableName){ case "users": $dg = new C_DataGrid("SELECT * FROM users", "id", "users"); $sdg = new C_DataGrid("SELECT * FROM contact", "id", "contact"); $dg->set_subgrid($sdg, 'sales_rep', 'id'); break; case "notes": $dg = new C_DataGrid("SELECT * FROM notes", "id", "notes"); break; case "contact": $dg = new C_DataGrid("SELECT * FROM contact", "id", "contact"); $sdg = new C_DataGrid("SELECT * FROM notes", "id", "notes"); $sdg->enable_edit(); $dg->set_subgrid($sdg, 'Contact', 'id'); break; } $dg->enable_edit(); $dg->display(); ?> |
My Sales Rep
Since a sales manager needs to quickly find out whom a sale person is working with, we added a detail grid $sdg populated from contact table and link with the parent grid.
1 2 | $sdg = new C_DataGrid("SELECT * FROM contact", "id", "contact"); $dg->set_subgrid($sdg, 'sales_rep', 'id'); |
sales_rep is the connecting key in contact table to the id that is the foreign key in users table. Remember the users stores all of our sales people information.
Screenshots
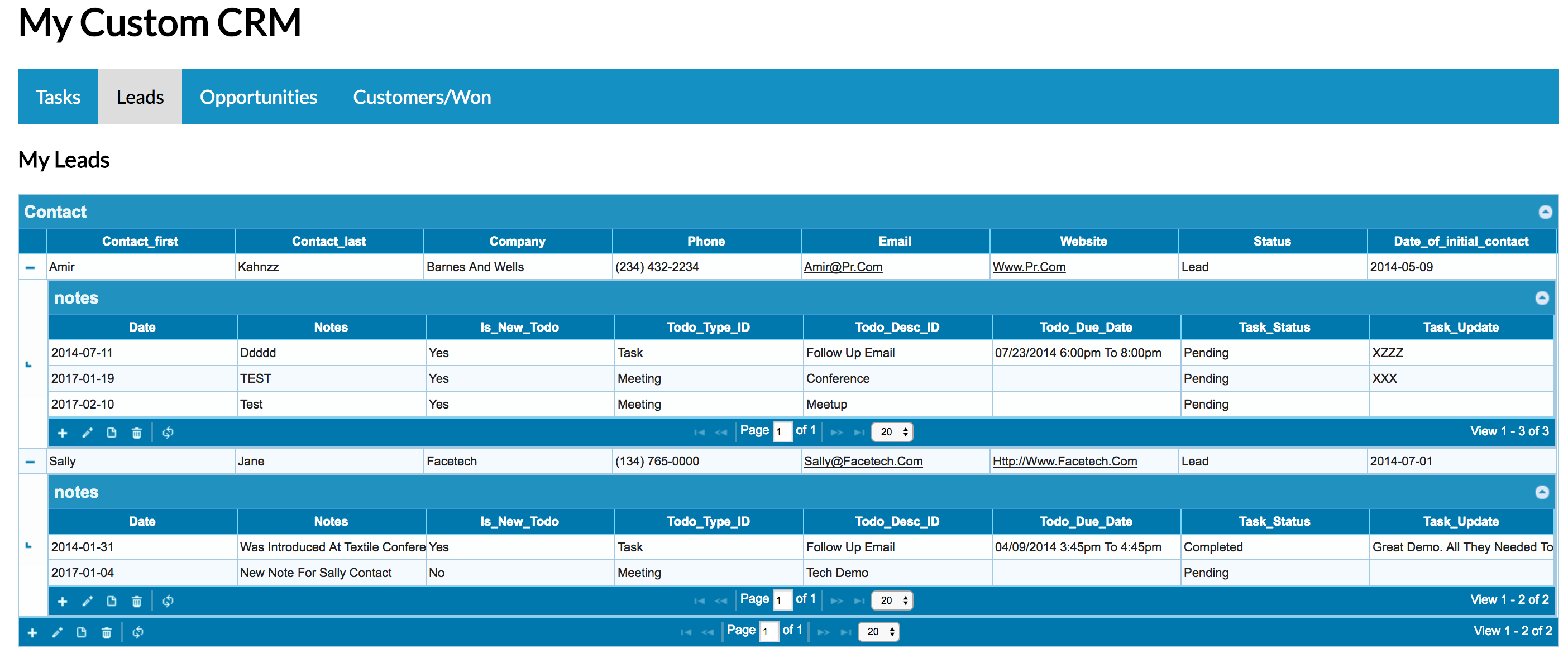
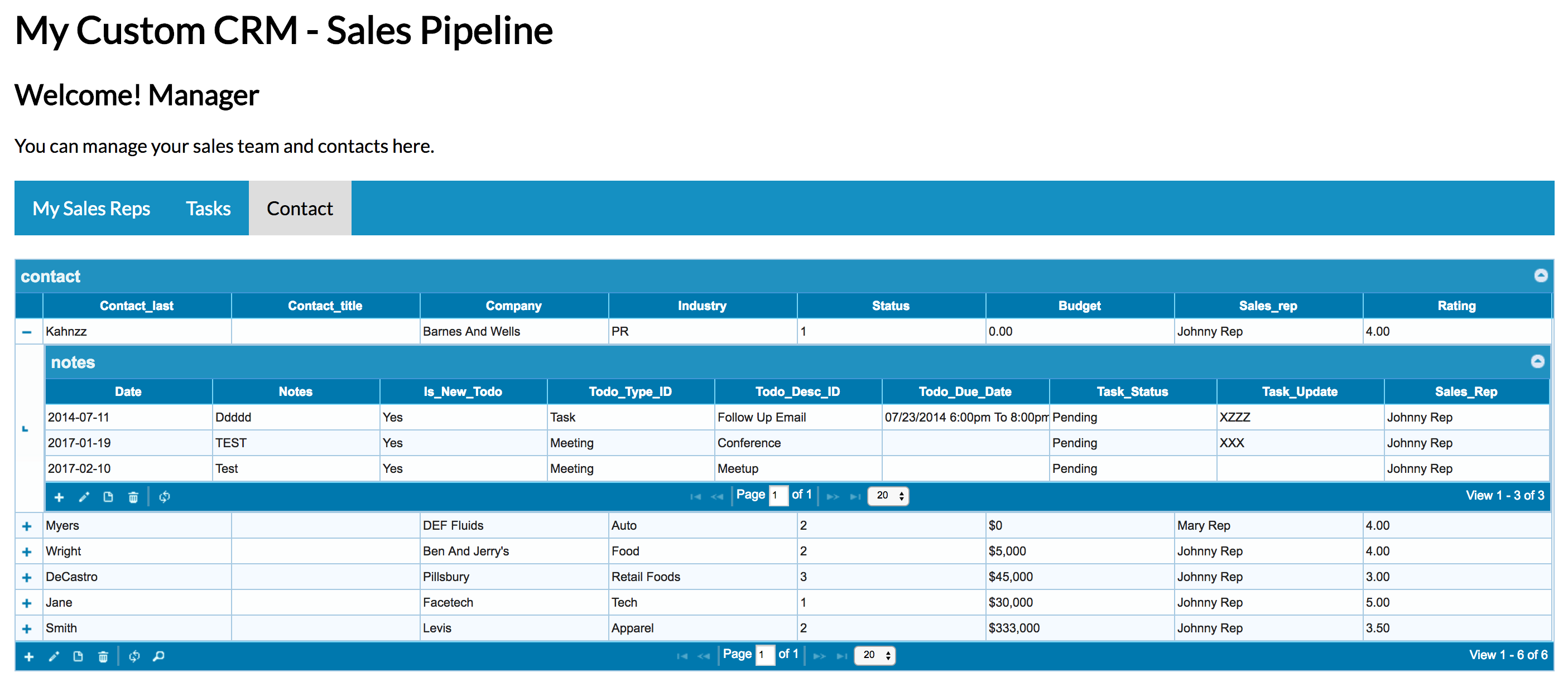
Interactive Demo
Login as Sales Rep Login as Manager Get this App ** Included in Ultimate edition.